In this following article we are going to see a simple code writing in selenium WebDriver using VS 2010.
Please set up environment for selenium webdriver in VS2010 with NUnit(you may see my previous post for that).
What the program will do ?
It will validate the tabs of an website. I am using http://www.kaz.com.bd/ for my example.
First, lets see the site. It has basic 4 tabs Home, Talents,Culture,Contact
To validate a tab we need
1. Is there any click event present?
2. Is there is any tab of that name?
3. Is the text (Name of the tab) is same as expected?
4. When we click, does the site goes to the expected destination?(after going there, the title will be loaded)
Step 1 : Launch VS 2010 and Add New Project Name : KazWebSite, Type : Class Library
Step 2 : Do the library & framework Integration to run web driver( see my previous post) and add a class Named “TestTabKAZ” and add include those libraries in the class(my previous post)
Step 3: Now write assign class as Test Fixture
Step 4 : Now , define WebDriver[we are using firefox, if you want, just change FirefoxDriver() it to InternetExplorerDriver()], Error logs, base URL
Step 5 : Define TestFixtureTearDown that will run one time after full test execution(Unload related tasks). In here we are quitting the driver and providing a notification to NUnit UI by assert exception if there is any error listed in error logs.
Step 6: Define a test case to test Home Tab. In Home tab of the site , we will test
-Title is ok or not(checking by assert string compare)
-Links is present or not(checking by assert true)
-URL link ok or not(checking by assert string compare)
-Home link spelling is ok or not(checking by assert string compare)
and If any error occurs, we will store into Error logs.
Note : In here, to get the element , we use IsElementPresent. It is a private method. For that, add the following code that will use Driver to find element and use NoSuchElementException.
Step 7 : Like as home tab, add test cases for “Talents”, “Culture”, “Contact” and finally the code will be like this
Step 8 : Now, build the solution (F6) [Don’t Run by F5 as it is not a executable project and it may show error message]
Step 9 : Open NUnit from Program file , Click Open Project and go to the stored location of the solution of VS project. Open Debug Folder.
Select the DLL named TestTabKAZ.dll
And Press Open.
Step 10: We will get NUnit GUI loaded with the project that we build. Please see the test cases that should be shown in GUI.
Step 11 : Running the Tests. We can run all tests together by selecting the Test Fixture and click Run or we can select an individual test case and Run the test.
If there is any Error, the NUnit GUI will show up with Error like this mentioning the text and which line the error occurs with the expected and actual values.
In , we have found the error with RED Bar mentioning error on line 100 as Expected the URL as cultural page where actual was contact page. From the code you can see , this occurs due to contact page load time delay for the Google Map present in contact page. These are real life issues. It may occur though you have written proper test cases. Now, from code , if we check URL at the last so that the page get time to be loaded, this error may not occur. And, its really is
We just change the following test part of culture.[testing URL at the end of other tests]
For the page load time, we may use time tracking code to measure load time and define failure cases for performance issues. In here, it will fail for a page if the time is more than 60s.
Now, we run an individual test case . Select TestHome and Run and see the results .
Here, the test pass with Green Bar.
So, In this small article, we have seen how to test simple tabs for a webpage. This part’s JAVA will be posted soon.
Thanks for staying with me….:)
Please set up environment for selenium webdriver in VS2010 with NUnit(you may see my previous post for that).
What the program will do ?
It will validate the tabs of an website. I am using http://www.kaz.com.bd/ for my example.
First, lets see the site. It has basic 4 tabs Home, Talents,Culture,Contact

To validate a tab we need
1. Is there any click event present?
2. Is there is any tab of that name?
3. Is the text (Name of the tab) is same as expected?
4. When we click, does the site goes to the expected destination?(after going there, the title will be loaded)
Step 1 : Launch VS 2010 and Add New Project Name : KazWebSite, Type : Class Library
Step 2 : Do the library & framework Integration to run web driver( see my previous post) and add a class Named “TestTabKAZ” and add include those libraries in the class(my previous post)
Step 3: Now write assign class as Test Fixture
[TestFixture]
public class TestTabKAZ
Step 4 : Now , define WebDriver[we are using firefox, if you want, just change FirefoxDriver() it to InternetExplorerDriver()], Error logs, base URL
private IWebDriver driver = new FirefoxDriver();
private StringBuilder errors=new StringBuilder();
private string baseURL = "http://www.kaz.com.bd";
Step 5 : Define TestFixtureTearDown that will run one time after full test execution(Unload related tasks). In here we are quitting the driver and providing a notification to NUnit UI by assert exception if there is any error listed in error logs.
[TestFixtureTearDown]
public void TeardownTest()
{
try
{
driver.Quit();
}
catch (Exception)
{
// Ignore errors if unable to close the browser
}
Assert.AreEqual("", errors.ToString());
}
-Title is ok or not(checking by assert string compare)
-Links is present or not(checking by assert true)
-URL link ok or not(checking by assert string compare)
-Home link spelling is ok or not(checking by assert string compare)
and If any error occurs, we will store into Error logs.
[Test]
public void TestHome()
{
driver.Navigate().GoToUrl(baseURL+"/index.html");
try
{
Assert.AreEqual("http://www.kaz.com.bd/index.html", driver.Url);
Assert.AreEqual("Home", driver.FindElement(By.LinkText("Home")).Text);//home link
Assert.AreEqual("Kaz Software", driver.Title);//title ck
Assert.IsTrue(IsElementPresent(By.LinkText("Home")));//element ck boolean
}
catch (Exception ex)
{
errors.Append("\n-Home Error");
errors.Append(ex.ToString());
throw ex;
}
}
private bool IsElementPresent(By by)
{
try
{
driver.FindElement(by);
return true;
}
catch (NoSuchElementException)
{
return false;
}
}
Step 7 : Like as home tab, add test cases for “Talents”, “Culture”, “Contact” and finally the code will be like this
using System;
using System.Text;
using System.Text.RegularExpressions;
using System.Threading;
using NUnit.Framework;
using OpenQA.Selenium;
using OpenQA.Selenium.Firefox;
using OpenQA.Selenium.Support.UI;
using OpenQA.Selenium.IE;
namespace KazWebSite
{
[TestFixture]
public class TestTabKAZ
{
private IWebDriver driver = new FirefoxDriver();
private StringBuilder errors = new StringBuilder();
private string baseURL = "http://www.kaz.com.bd";
[TestFixtureTearDown]
public void TeardownTest()
{
try
{
driver.Quit();
}
catch (Exception)
{
// Ignore errors if unable to close the browser
}
Assert.AreEqual("", errors.ToString());
}
[Test]
public void TestHome()
{
driver.Navigate().GoToUrl(baseURL + "/index.html");
try
{
Assert.AreEqual("http://www.kaz.com.bd/index.html", driver.Url);
Assert.AreEqual("Home", driver.FindElement(By.LinkText("Home")).Text);//home link
Assert.AreEqual("Kaz Software", driver.Title);//title ck
Assert.IsTrue(IsElementPresent(By.LinkText("Home")));//element ck boolean
}
catch (Exception ex)
{
errors.Append("\n-Home Error");
errors.Append(ex.ToString());
throw ex;
}
}
[Test]
public void TestTalents()
{
driver.Navigate().GoToUrl(baseURL + "/talent.html");
//driver.FindElement(By.LinkText("Talents")).Click();
try
{
Assert.AreEqual("Kaz Software - Talents", driver.Title);
Assert.AreEqual("Talents", driver.FindElement(By.LinkText("Talents")).Text);
Assert.IsTrue(IsElementPresent(By.LinkText("Talents")));
Assert.AreEqual("http://www.kaz.com.bd/talent.html", driver.Url);
}
catch (Exception ex)
{
errors.Append("\n-Talents Error");
errors.Append(ex.ToString());
throw ex;
}
}
[Test]
public void TestCulture()
{
driver.Navigate().GoToUrl(baseURL + "/culture.html");
//driver.FindElement(By.LinkText("culture")).Click();
try
{
Assert.AreEqual("http://www.kaz.com.bd/culture.html", driver.Url);
Assert.AreEqual("Kaz Software - Culture", driver.Title);
Assert.IsTrue(IsElementPresent(By.LinkText("Culture")));
Assert.AreEqual("Culture", driver.FindElement(By.LinkText("Culture")).Text);
}
catch (Exception ex)
{
errors.Append("\n-Culture Error");
errors.Append(ex.ToString());
throw ex;
}
}
[Test]
public void TestContact()
{
driver.Navigate().GoToUrl(baseURL + "/contact.html");
//driver.FindElement(By.LinkText("contact")).Click();
try
{
Assert.AreEqual("http://www.kaz.com.bd/contact.html", driver.Url);
Assert.AreEqual("Kaz Software - Contact", driver.Title);
Assert.IsTrue(IsElementPresent(By.LinkText("Contact")));
Assert.AreEqual("Contact", driver.FindElement(By.LinkText("Contact")).Text);
}
catch (Exception ex)
{
errors.Append("\n-Contact Error");
errors.Append(ex.ToString());
throw ex;
}
}
private bool IsElementPresent(By by)
{
try
{
driver.FindElement(by);
return true;
}
catch (NoSuchElementException)
{
return false;
}
}
}
}
Step 9 : Open NUnit from Program file , Click Open Project and go to the stored location of the solution of VS project. Open Debug Folder.
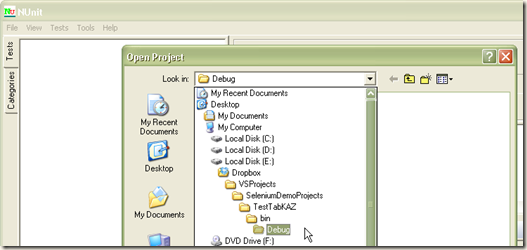
Select the DLL named TestTabKAZ.dll

And Press Open.
Step 10: We will get NUnit GUI loaded with the project that we build. Please see the test cases that should be shown in GUI.

Step 11 : Running the Tests. We can run all tests together by selecting the Test Fixture and click Run or we can select an individual test case and Run the test.
If there is any Error, the NUnit GUI will show up with Error like this mentioning the text and which line the error occurs with the expected and actual values.
In , we have found the error with RED Bar mentioning error on line 100 as Expected the URL as cultural page where actual was contact page. From the code you can see , this occurs due to contact page load time delay for the Google Map present in contact page. These are real life issues. It may occur though you have written proper test cases. Now, from code , if we check URL at the last so that the page get time to be loaded, this error may not occur. And, its really is
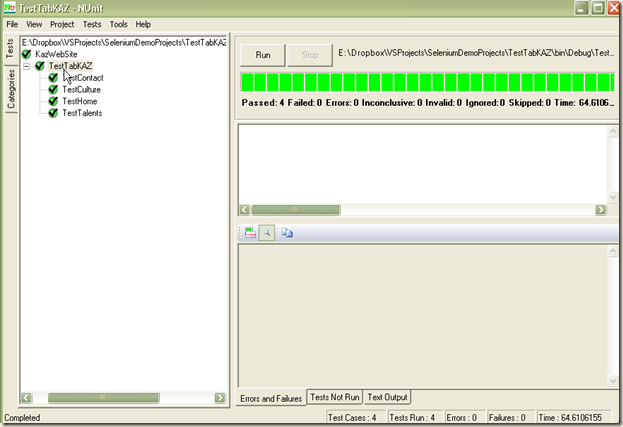
We just change the following test part of culture.[testing URL at the end of other tests]
Assert.AreEqual("Kaz Software - Culture", driver.Title);
Assert.IsTrue(IsElementPresent(By.LinkText("Culture")));
Assert.AreEqual("Culture", driver.FindElement(By.LinkText("Culture")).Text);
Assert.AreEqual("http://www.kaz.com.bd/culture.html", driver.Url);
For the page load time, we may use time tracking code to measure load time and define failure cases for performance issues. In here, it will fail for a page if the time is more than 60s.
if (second >= 60)
Assert.Fail("timeout");
Now, we run an individual test case . Select TestHome and Run and see the results .
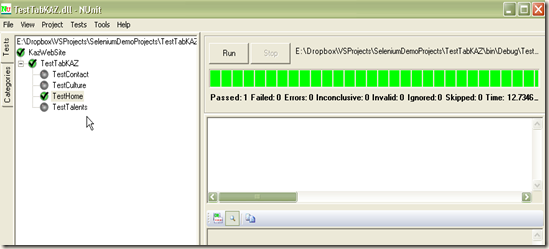
Here, the test pass with Green Bar.
So, In this small article, we have seen how to test simple tabs for a webpage. This part’s JAVA will be posted soon.
Thanks for staying with me….:)